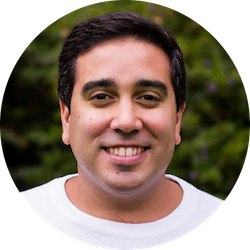
By Juan Andrés
Check out the fourth edition of our React newsletter:
React and animations
There are several libraries that are extremely useful for adding animation to components, some of the most popular are:
react-motion: This library allows defining of animation parameters using physical aspects. For example, when animating a spring, one can define the stiffness or the damping of it. This provides enormous help in creating more realistic and natural animations.
react-move: A small library (12k) that provides extremely fine tuning for animation parameters.
velocity-react: For interacting with Velocity.js using React.
react-gsap-enhancer: A React component enhancer for applying GSAP animations on components without side effects. This may be too much for simple animations, but can prove useful for more complex scenarios.
react-flight: Animation library that prioritizes simplicity and ease of use. It also has integration with Redux so that animations can be performed depending on the state.
React, Apollo and GraphQL
When developing a website that interacts with an REST API, a common path is to use a network management library together with Redux to manage state. This means writing reducers, actions, and state management for the different stages when communicating with the API. With good design, this can be generalized using HOCs, or some other structure to avoid repeating code.The Apollo stack aims to simplify development of applications that consume an API.
GraphQL
For the backend part of the stack, it uses GraphQL instead of REST. GraphQL is a query language created by Facebook in 2012 for describing the capabilities and requirements of data models for client‐server applications. It tackles several of REST's shortcomings, providing an easy way of defining data structures and queries so that development and usage of the API is easy and flexible. Some people are even calling it REST 2.0.
There is a whole ecosystem around GraphQL. Some notable examples of tools are:
GraphCool provides a way to use GraphQL like a backend-as-a-service.
Plugins exists to validate queries using ESLint, avoiding typos and warning the developer if something changed in the backend.
Some are pointing to GraphiQL (an interactive tool for exploring and testing GraphQL backends) as the killer app for GraphQL.
Apollo
Apollo is a GraphQL client for React, React Native, Angular, Swift and Java. When using it, you get options for configuring caching, mutations, optimistic UI, subscriptions, pagination, server-side rendering, prefetching, and more.
It can be incrementally adopted, meaning that it can be implemented for small components, coexisting with non-apollo components. Apollo uses Redux under the hood, so it can plug into existing Redux applications and all Redux development tools will work with Apollo's queries.
All code related to API interactions gets managed by Apollo, allowing the developer to just define what data a component needs.
Besides the official documentation, these are some useful resources:
Full-stack React + GraphQL Tutorial: an excellent 6-part tutorial for implementing the full Apollo stack.
How do I GraphQL?: Top 3 things to know coming from REST.
GraphQL explained: How GraphQL turns a query into a response.
Simplify your React components with Apollo and Recompose: Keeping your components pure with HoCs
Tools
https://ngrok.com/: Secure tunnels to localhost. Solves the problem: ”I want to expose a local server behind a NAT or firewall to the internet.” Extremely useful for demos, and scenarios where a quick exposition of localhost to the internet is useful instead of a deployment. It also provides a dashboard to inspect all incoming and outgoing traffic.
styled-components/primitives: Creating truly universal React components that can be rendered on the web and in React Native is hard. There are different primitives:<View> vs. <div>,<Text> vs. <h1>/<p> etc, and the styling also works entirely differently: ReactNative has it’s own CSS-like styling solution with JS objects, StyleSheet.
Leland Richardson (Airbnb) has been working to create a shared set of primitives to be used to build truly cross-platform React apps. It’s called react-primitives and it’s a collection of six core components that are all you need to build everything you can dream of and render them consistently across the web, native and even Sketch.
Code splitting in create-react-app: Now supported by create-react-app, dynamically import and load parts of a React application instead of loading the whole JavaScript.