
By Juan Andrés
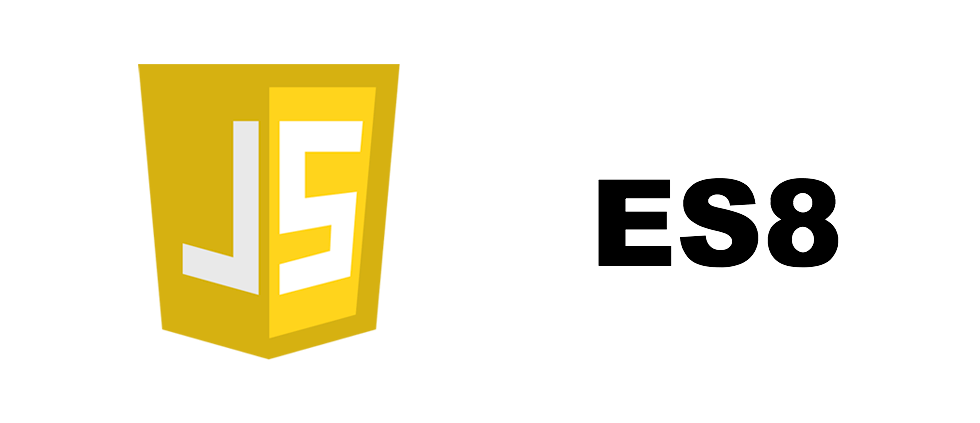
Javascript ECMAScript 2017 (ES8)
Some cool features that are introduced in ES8:
Async/Await
As Javascript developers, managing asynchronous code is a common task. Sometimes complex logic must be written around it to manage error handling, variables and callbacks. Async/Await is a way to make developers' lives easier. It can achieve the same results as code written using promises but with much better readability and clarity.A good and clear list of reasons why using Async/Await is a good idea can be found in this excellent article: 6 Reasons Why JavaScript’s Async/Await Blows Promises Away
Object.values and Object.entries
The Object.values method returns an array of a given object’s own enumerable property values.The Object.entries method returns an array of a given object's own enumerable property [key, value] pairs, in the same order as Object.values

Debugging React
The React ecosystem allows developers to integrate a large number of tools to their workflow. This flexibility allows for increased productivity and avoids being locked into a single vendor. The downside is that having such a partitioned approach sometimes obscures information flow and makes debugging more difficult.Several tools exist to improve the debugging experience, and having knowledge on how to use them has a positive impact on productivity and bug fixing speed.ESLint, the JavaScript Console, the browsers' inspect window (together with their source code and networking tabs), React and Redux Developer tools, and many other can give in-depth insights into the inside workings of your application.This article explains most of them and how to make good use of them: Intro to debugging ReactJS applications
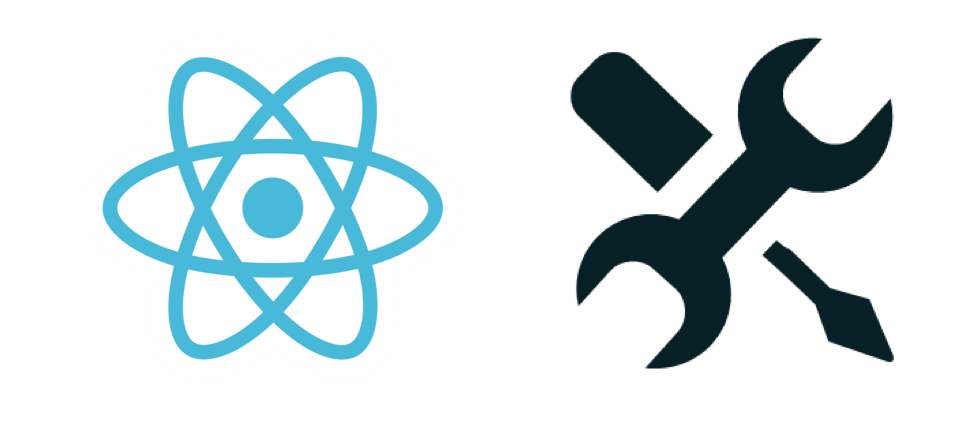
Tools
- How to GraphQL: Free and open-source tutorial to learn all about GraphQL. Go from zero to production. It contains sections for combining React, Vue, Apollo, Relay and many more.
- BlueprintJS: Yet another React UI toolkit. It doesn't have mobile support, but it has a large number of controls for creating complex desktop applications.
- React Loadable: Component-centric code splitting and loading in React. This can improve loading times dramatically and it doesn't need too much effort to implement.