Core Data is a powerful framework for managing and persisting data in iOS applications. It provides an object-oriented approach to working with data, allowing developers to interact with their data using familiar concepts like objects and relationships. In this article, we'll explore what Core Data is, why it's beneficial for iOS development, and how to set it up in your project. We'll also cover how to perform CRUD (Create, Read, Update, Delete) operations with Core Data.
What is Core Data and Why Use It?
Core Data is an Apple framework designed to handle the model layer in your applications. It's not just a database; it's an entire object graph and persistence framework that provides several benefits:
1. Data Management: Core Data helps manage the life cycle of your data objects, including efficient memory management and versioning.
2. Persistence: Easily save and retrieve data to and from a persistent store (e.g., SQLite database).
3. Undo and Redo: Built-in support for undo and redo functionality.
4. Data Model Relationships: Define complex relationships between your data objects, which Core Data manages efficiently.
5. Data Migration: Smoothly handle changes to your data model over time with minimal effort.
Core Data is an excellent choice for mobile development because it allows you to manage your app's data efficiently and robustly, ensuring a smooth and responsive user experience.
Setting Up Core Data in Your Project
Integrating Core Data into your iOS project is straightforward. Follow these steps to set up Core Data in your Xcode project:
1. Create a New Project:
- Open Xcode and create a new project.
- Select "App" under the iOS tab and click "Next."
- Enter your project details, and ensure the "Use Core Data" checkbox is checked. This will automatically include the necessary Core Data setup in your project.
2. Core Data Stack:
- Xcode will generate a `CoreDataStack` class or similar utility that sets up the Core Data stack, including the `NSPersistentContainer`, which manages the Core Data stack.
3. Data Model:
- Xcode also creates a `.xcdatamodeld` file, which is where you define your data model. Open this file and add your entities, attributes, and relationships.
Performing CRUD Operations with Core Data
Once you have set up Core Data in your project, you can perform CRUD operations. Let's explore each operation:
Create
To create a new record, you must create a new managed object and save it to the context.

Read
To fetch data from Core Data, you use a NSFetchRequest.

Update
Updating a record involves fetching it first, then modifying its attributes and saving the context.

Delete
To delete a record, fetch it first and then delete it from the context.
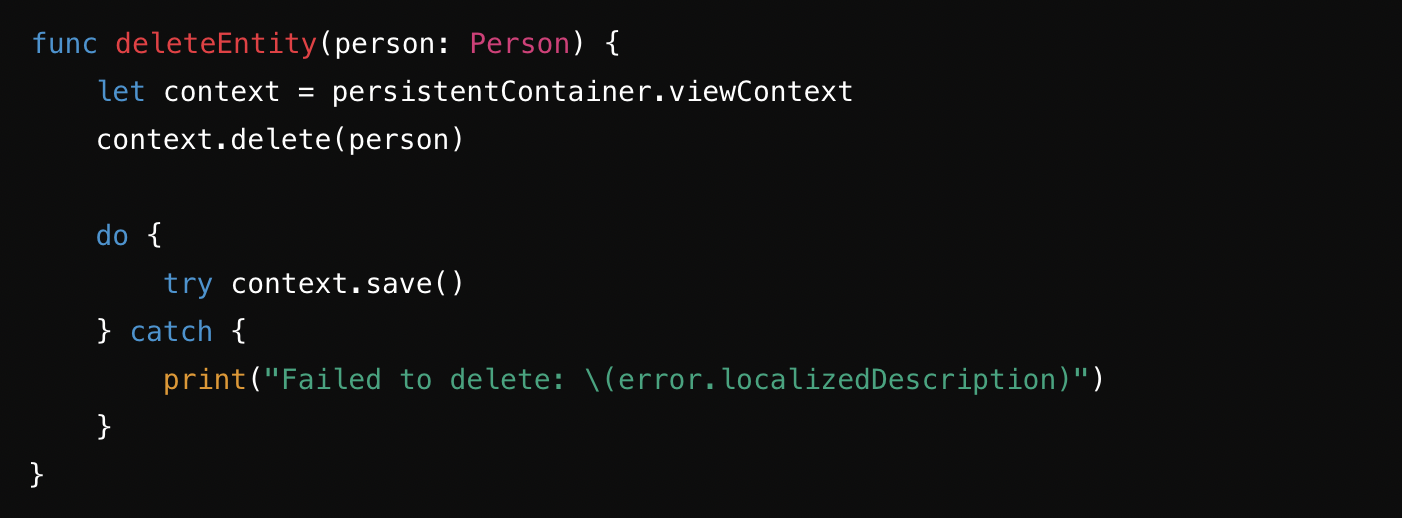
Conclusion
Integrating Core Data into your iOS app is a great way to manage and persist data efficiently. With its robust features and seamless integration into the iOS ecosystem, Core Data simplifies data management in mobile development. By following the steps outlined in this article, you can set up Core Data in your project and perform essential CRUD operations, enhancing your app's functionality and user experience.
By mastering Core Data, you elevate your iOS development skills, ensuring your apps are powerful and efficient in handling data. Happy coding!